Nowadays, users are presented with various inputs in the website, which when interacted with, changes the existing UI of the website directly or indirectly via executing some JavaScript.
Almost all the sites are dynamic today, which contains some sort of logic in JavaScript script files or code present between <script>…</script> tags which can be inserted anywhere in the document.
XSS - Cross Site Scripting is nothing but injecting malicious JavaScript in trusted websites that is executed on the visitor’s browser.
An example
Lets take an example where in a form is presented to the user which asks for the user’s name and when submitted, opens up another page with the name being set as the heading of the page.
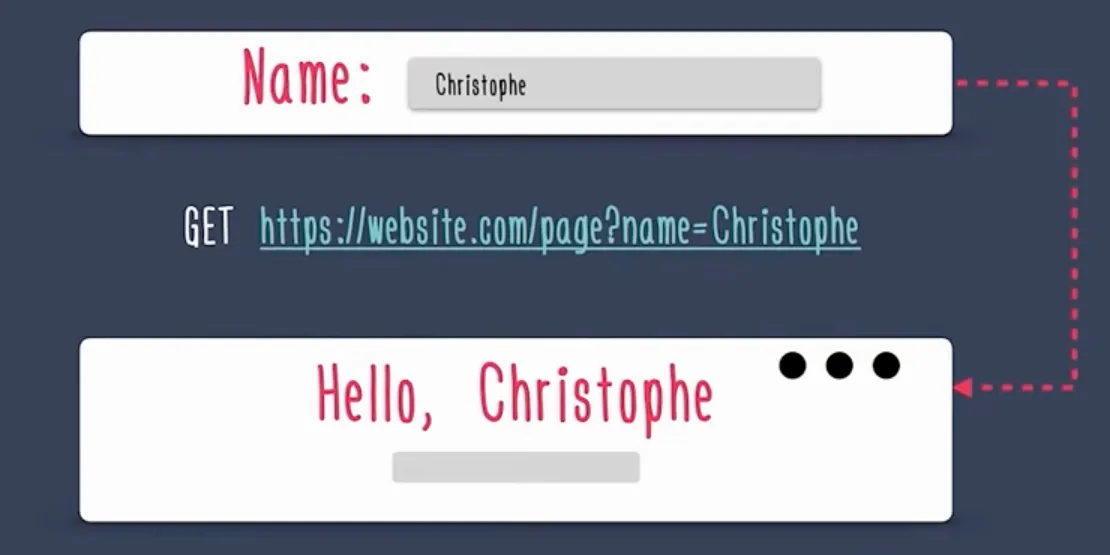
Considering developers have not sanitized (encoded) the input received in the input box, and has rendered it onto the page, any hacker can easily inject his/her own script by simply adding a script via script tags in the input itself.
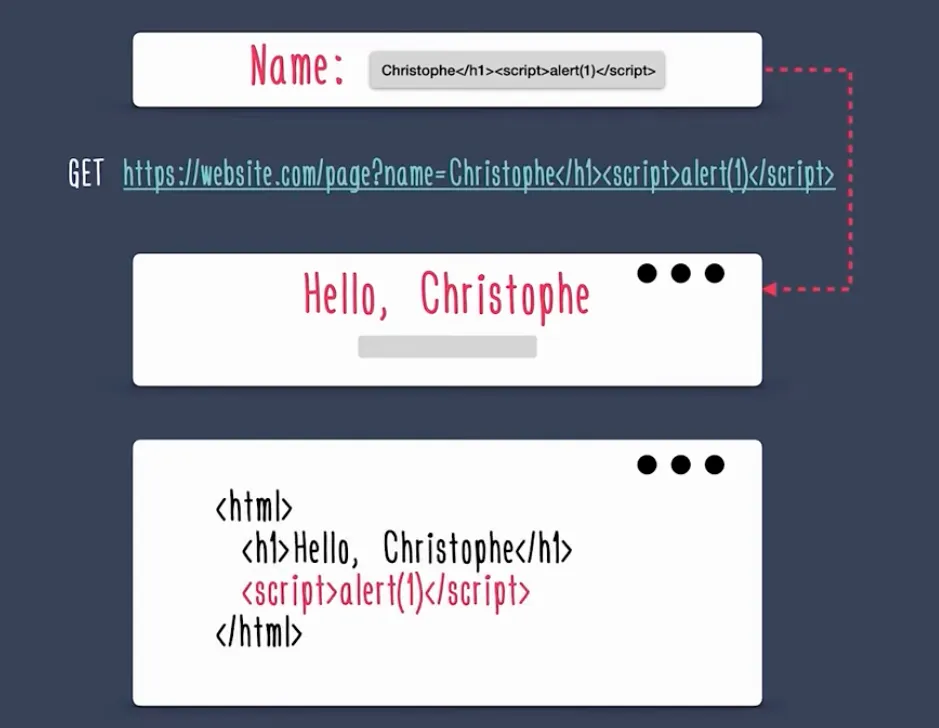
Just by adding Christophe</h1><script>alert(1)</script>
in the input, the URL of the redirected page now turned to
https://website.com/page?name=Christophe</h1><script>alert(1)</script>
which then tried to render it as it was in the HTML doc which now contains the malicious script passed in the query param.
This script can come from anywhere, and might contain some event listeners which are listening to the keystrokes that you are making while filing a very important form, or can access some sensitive information provided by you.
Not just a script, but an entire form can be injected in this way, as it’ll get rendered as it is in the page and might ask user to put in some sensitive data.
Reflected vs Persisted vs DOM Based attack
A reflected attack is actually the scenario which is mentioned above wherein the attacker doesn’t necessarily infect the server, but uses it to serve malicious script to the visitor which gets executed in the visitor’s browser itself.
A persisted attack is the same as reflected, the only difference being the malicious data is now stored in a database and then accessed by the visitor. This again doesn’t mean that the server is infected.
A DOM based attack is a scenario wherein the servers aren’t even involved! The malicious script gets added to the DOM via the input itself without sending it to a server.
Encoding
XSS is only possible when the application uses the input provided by the user within the output without properly validating or encoding the data.
Encoding is nothing but converting the input or the sequence of characters in a specific format which can be used to process the data securely.
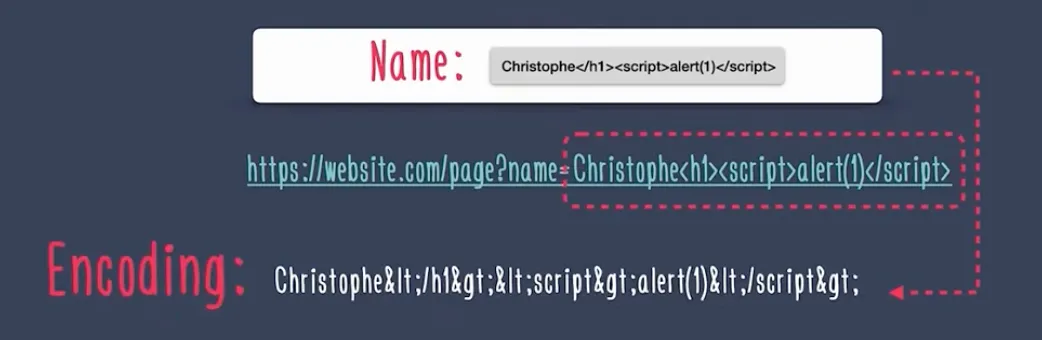
CSRF - Cross Site Request Forgery
A bit of background
Mostly, authentication and authorization works with the help of session cookies, wherein a session cookie is generated as soon as someone logs in and is sent back to the client which then passes it along with every further HTTP requests.
There are many tools provided to safeguard these cookies -
- secure flag - which when true, enables the cookie to be sent only for https domains.
- httpOnly flag - which when true, enables the cookie to only be accessed via HTTP calls, and not via some JavaScript script.
- CORS - which restricts other origins or sites to make a request to this server.
However, there is a limitation of CORS - It doesn’t stop FORM actions from other origins or sites to make a call to the server.
When does CSRF occurs
It occurs when any foreign site makes request to the server via FORM actions or some other way -
<form action="<some HTTP endpoint of your server>"></form>
An example would be the endpoint to delete a USER which just relies on the UUID being sent as incoming session cookie, gets the user marked against that UUID and then deletes both the UUID and the user from the database.
If another site places a form action pointing to the deletion endpoint, while the user is authenticated (UUID is stored in cookies), then the account of that user can get potentially deleted if extra validation is not applied.
The same concept can be utilized for accessing different endpoints, which does different functions.
Solution for CSRF
Another token called csrfToken can be generated and tracked for the user along with the session UUID at the server end. Upon receiving this csrfToken, client can essentially save it in the code itself where its not exposed to any other entity.
This csrfToken can be passed along in the body of the requests for which we want to have this extra validation. And an extra check can be placed for this csrfToken at the server, which will ensure that the entity making this request is the correct one.